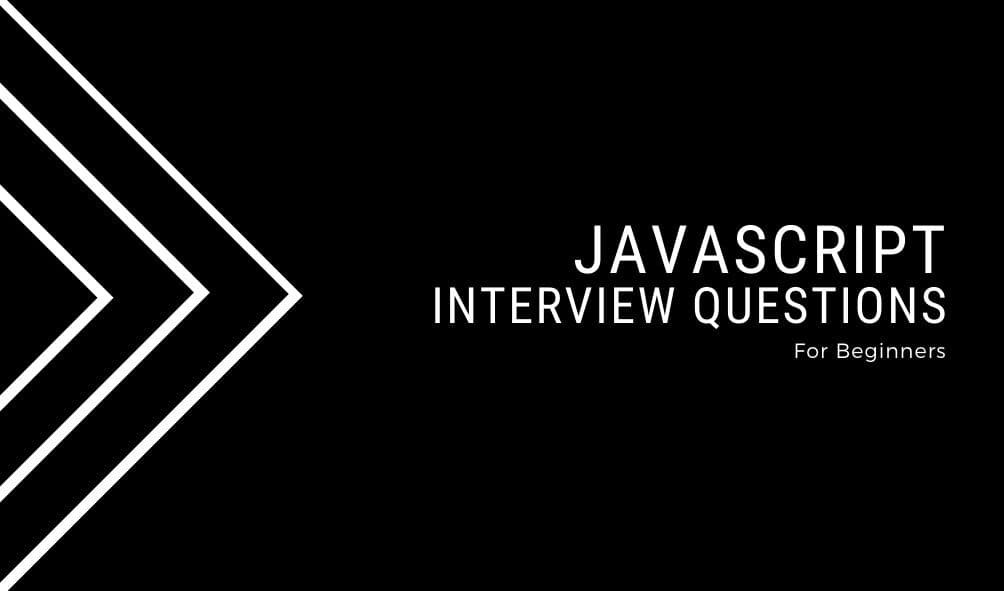
Here is the list of frequently asked JavaScript interview questions for beginners with answers. You can read and give feedback if you like it.
What is JavaScript?
JavaScript is a scripting language that is used to interact with the web browser for dynamically updating content, animations, handing multimedia , events etc. It is high level , lightweight, interpreted and just in time compile language.
What does JavaScript do?
- It is used to implement dynamic behavior on web page.
- It has some common programming features such as variables, functions, array,, loop etc.
- It is used to handle events.
What is the difference between ECMAScript and JavaScript?
ECMAScript( European Computer Manufacturer’s Association ) provides Standard for scripting languages such as JavaScript, JScript, etc. It is a trademark scripting language specification. JavaScript is a language based on ECMAScript
Why JS include at the bottom of page?
JavaScript is included at the bottom of page because so that page load time will not increase. Also DOM will be loaded first then the JavaScript will be loaded.
What is a variable?
Variable is used to store data temporarily.
Rules to create a variable
It cannot be reserved keyword
It should be meaningful
It cannot start with number
It cannot have space or hyphen
It is case sensitive.
var num = 10; // here num is a variable
By Default variables in JavaScript are?
0
Null
undefined
undefined
What is Constant?
As its name suggest constant value never changed. It remains constant throughout the program. If constant value has been changed, it through error.
const pi = 3.14; // a constant
if value of constant is changed to something else
pi = 3.141 //gives TypeError: invalid assignment to const `pi'
What if you re-declare or re-assign constant value?
It will gives Type error invalid assignment
How to clear the value of a variable in JavaScript?
By assigning it to null
Difference between null and undefined in JavaScript?
They both are falsy values however they are different from each other because of following
Null means nothing while undefined means a variable is declared but no value is assigned to it.
var a; //undefined var c = null; // null
Type of null is object while type of undefined is undefined.
var a; console.log(typeof(a)); //undefined var c = null; console.log(typeof(c)) // object
What is Object in JavaScript?
In JavaScript objects are variables that store the data in key value pair.
var book = {title:"Twilight Saga", author:"Stephenie Meyer", published_date:"Jan 2005"}; //JavaScript object
How to create JavaScript object and access it?
<script> let pen = { color : 'red', model : 'celo' }; console.log(pen); // object console.log(pen.color); // red using dot notation console.log(pen['model']); // using bracket notation </script>
How to insert value into an existing JavaScript object?
using dot notation
let pen = { color : 'red', model : 'celo' }; pen.price = 5; //using dot notation
using bracket notation
let pen = { color : 'red', model : 'celo' }; pen['price'] = 5;
Explain for in loop in JavaScript
for in loop is used to traverse the objects.
<script> let pen = { color : 'red', model : 'celo' }; for (key in pen){ console.log(key); // will print color model console.log(key + " : " + pen[key]); //wil print color red model celo } </script>
According to MDN
Note: for...in
should not be used to iterate over an Array
where the index order is important.
Explain for of loop in JavaScript.
It introduced in ES6 . Using this loop values can be printed directly.It is used to iterate over array.
<script> let pen2 = ['red','celo']; let pen = { color : 'red', model : 'celo' }; for (key of Object.keys(pen)){ // objects are not iterable directlt using for of loop console.log(key); // will print red celo } for (key of pen2){ console.log(key); // will print red celo } </script>
Refer Here for Object.keys
What is arrays in JavaScript?
Array is a variable that can store multiple values. In JavaScript neither length of array nor type of element of array is fixed.
<script> /// to declare an array let colors = ['red','blue']; // to access array values colors[2] = 'black'; colors[3] = 1; console.log(colors); console.log(colors[0]); </script>
What does typeof operator do ?
This tells the type of a variable. For example whether it is string, number, null, undefined, array, object
How do you determine length of array?
<script> let colors = ['red','blue']; colors[2] = 'black'; console.log(colors.length); </script>
What are functions in JavaScript?
Functions are piece of code that can be reused later in a program.
<script> function sayHello(name){ // name is parameter let str = "Hello"+ ' ' + name; console.log(str); return str; } sayHello("jass"); // jass is an argument </script>
What are expression in JavaScript?
Any statement of code that can be evaluated into a value is called expression.
let sum = 7+9; //Arithmetic Expression 20 > 10 // logical expression "curious" + "webs" // string expression sum+= 1 // assignment expression
What is the difference between “===” and “==” operator?
“===” compare type and value while ” ==” compare value only
<script> console.log(1==1); //returns true console.log('1'==1); //returnsn true console.log(1===1); //returns true console.log('1'===1); //returnsn false </script>
Write syntax of switch case statement in JavaScript
switch(expression) { case a: // code block break; case b: // code block break; default: // code block }
What do you mean by NaN in JavaScript?
NaN means not a number. This just tells whether there is legal numerical value or not. isNan() is used to check whether the number is not a number or string.
isNaN(3) //false isNaN(-1) //false isNaN(5+4) //false isNaN('Hello') //true isNaN('2005/12/12') //true isNaN('') //false isNaN(true) //false isNaN(undefined) //true isNaN('NaN') //true isNaN(NaN) //true isNaN(0 / 0) //true
What does Math.floor function do in JavaScript?
Math.floor() returns the largest integer from given value.
Math.floor(2.5); // return 2 Math.floor(2.1); //return 2 Math.floor(2); //return 2
What is factory functions in JavaScript?
Factory functions returns object without using new keyword. It is neither a class nor a constructor.
function sayHello(name) { return { name : name, // it means name:name hello() { console.log("Hello",name); } }; } const sayhello = sayHello("World"); console.log(sayhello); console.log(sayhello.name); console.log(sayhello.hello());
What is constructor functions in JavaScript?
In constructor function object is created using new keyword. The properties of function initialized using this keyword.
function sayHello(name) { this.name = name, // it means radius:radius this.hello = function(name) { console.log("Hello",this.name); } } const sayhello = new sayHello("World"); console.log(sayhello); console.log(sayhello.name); console.log(sayhello.hello());
What is this keyword in JavaScript?
this keyword in JavaScript refer to the object that is executing the code.
Refer here for more information
What is the new keyword in JavaScript?
“new” keyword in JavaScript creates a blank object and link this object to another object and passes the newly created object as this context. It returns this
if the function doesn’t return its own object.
function sayHello(name) { this.name = name, // it means radius:radius this.hello = function(name) { console.log("Hello",this.name); } } const sayhello = new sayHello("World"); console.log(sayhello.name); console.log(sayhello.hello());
By default this refers to window object.
What do you mean objects are dynamic in nature?
Dynamic nature of object means property of object can be set during runtime.
const book = { author: "stephene", edition: "1.1" }; book.releaseDate = "05-05-2005"; //adding property to objects book.chapters = function() {}; console.log(book);
What is delete operator in JavaScript?
This operator delete the property and its value.
const book = { author: "stephene", edition: "1.1" }; delete book.author; // output : undefined
In JavaScript functions are objects. Explain.
Functions are object because they have properties and method just like an object.
function sayHello(name) { this.name = name, // it means radius:radius this.hello = function(name) { console.log("Hello",this.name); } } const sayhello = new sayHello("World");
How to convert object into array in JavaScript.
in ES6 using three ways
const name = {
first_name: "Stephene",
last_name: "Rodger"
};
Object.keys(name) // [
'first_name', 'last_name']
Object.values(name) // ['Stephene', 'Rodger']
Object.entries(name) // [ ['first_name', 'Stephene'], ['last_name', 'Rodger'] ]
How to check if property exist in JavaScript object
using .hasOwnProperty() method and if in block
const object1 = new Object(); object1.property1 = 42; console.log(object1.hasOwnProperty('property1'));
if ('prop' in obj) { // do something }
How you copy one object into another?
By using spread operator
var obj1 = { foo: "foo", bar: "bar" };
var copiedObj= { ...obj1 }; //
copiedObj{ foo: "foo", bar: "bar" }
By using object.assign() method
var obj1 = { foo: "foo", bar: "bar" };
var copiedObj=
Object.assign({}, obj);
// copiedObj{ foo: "foo", bar: "bar" }
The only problem with above method is they create a shallow copy. It means if object1 has object reference and you create a copy of it and then if changes were made into the object of copied object then the changes will be reflect into the object1 too. Here is example
var obj1 = { foo: "foo", bar: "bar", c : {test:"test_obj"} };
var copiedObj=
Object.assign({}, obj);
// copiedObj{ foo: "foo", bar: "bar",{test:"test_obj"}}
// now make changes in copiedObj copiedObj.c.test = "test_data"; console.log(copiedObj); console.log(obj1);
To avoid this JSON.stringify
function is used because it only copies the reference value
What is spread operator in JavaScript?
(…) spread operator is used to iterate over an array,string or object.
const arr1 = [0, 1, 2];
const arr2 = [3, 4, 5];
const arr3 = [...arr1,...arr2];
Any JavaScript math function
Math.random() : Generate random number
Math.round() : returns the value of a number rounded to the nearest integer.
console.log(Math.round(0.9)); // expected output: 1
Math.max() : returns the largest of zero or more numbers.
console.log(Math.max(1, 3, 2)); // expected output: 3
Math.min() : returns the lowest-valued number passed into it, or NaN
if any parameter isn’t a number and can’t be converted into one
console.log(Math.min(-2, -3, -1)); // expected output: -3
Math.sqrt() : returns the square root of a number
console.log(Math.sqrt(9)); // expected output: 3
How to declare a string as object in JavaScript?
By using String Constructor
<script> var objectString = new String('Hello World'); console.log(typeof(objectString)); // object </script>
Name some JavaScript string function.
string.length() : returns the length of a string
const str = 'This is a string having length is:'; console.log(str + ' ' + str.length); // output : This is a string having length is: 34
string.include(): checks whether the sub-string is included in a string or not.
const sentence = 'The quick brown fox jumps over the lazy dog.';
const word = 'fox';
console.log(The word "${word}" ${sentence.includes(word)? 'is' : 'is not'} in the sentence
);
string.startsWith() : check whether string ends with specified character
const str2 = 'Is this a question'; console.log(str2.startsWith('Is')); // expected output: true
string.endsWith() : check whether string ends with specified character
const str2 = 'Is this a question'; console.log(str2.endsWith('?')); // expected output: false
string.indexOf() : returns the first occurrence of matched string. -1 if not found.
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?'; const searchTerm = 'dog'; const indexOfFirst = paragraph.indexOf(searchTerm);
How to replace string in JavaScript?
By using string.replace
const p = 'The quick brown fox jumps over the lazy dog. If the dog reacted, was it really lazy?'; const regex = /dog/gi; console.log(p.replace(regex, 'ferret'));
How to trim a string in JavaScript?
By using string.trim() method. It trim white-space( (space, tab, no-break space, etc. ) from both ends of a string.
const greeting = ' Hello world! '; console.log(greeting.trim());
What is escape Notation in JavaScript
new line chracter \n
How to convert a string into array in JavaScript?
By using string.split()
const str_ = 'String'; console.log(str_.split(''));
What is template literals in JavaScript?
template literals are string literals allowing embedded expressions.
`string text ${expression} string text`
let a = 5; let b = 10; console.log(`Fifteen is ${a + b} and not ${2 * a + b}.`);
Name some data function in JavaScript.
Date() : It gives the current date time and day
console.log(Date()) // Sat Mar 21 2020 10:32:52 GMT+0530 (India Standard Time)
getFullYear () : returns the year
let d = new Date(); console.log(d.getFullYear()); // output : 2020
getMonth() : returns numeric value of month
var d = new Date(); var months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; document.getElementById("demo").innerHTML = months[d.getMonth()];
Name some array function JavaScript.
splice() : used to insert value in array
const months = ['Jan', 'March', 'April', 'June']; months.splice(1, 0, 'Feb'); // inserts at index 1 console.log(months); // expected output: Array ["Jan", "Feb", "March", "April", "June"]
indexOf() : returns the index of the element that is first found in a given array. It returns -1 if not present.
const beasts = ['ant', 'bison', 'camel', 'duck', 'bison']; console.log(beasts.indexOf('bison'));
push() : used to push value in an array
const animals = ['pigs', 'goats', 'sheep']; const count = animals.push('cows'); console.log(animals); // output : ['pigs', 'goats', 'sheep', 'cows']
unshift() : add element at the beginning of array()
const array1 = [1, 2, 3]; console.log(array1.unshift(4, 5)); // expected output: Array [4, 5, 1, 2, 3]
includes() : whether an array includes a certain value among its entries and returns boolean value.
const array1 = [1, 2, 3]; console.log(array1.includes(2)); // expected output: true
find() : return the value of first element that is found
const array1 = [5, 12, 8, 130, 44]; const found = array1.find(element => element > 10); console.log(found);
pop() : pops out the last element
const plants = ['broccoli', 'cauliflower', 'cabbage', 'kale', 'tomato']; console.log(plants.pop()); // expected output: "tomato" console.log(plants); // expected output: Array ["broccoli", "cauliflower", "cabbage", "kale"] plants.pop(); console.log(plants);
concat() : used to merge two arrays.
const array1 = ['a', 'b', 'c']; const array2 = ['d', 'e', 'f']; const array3 = array1.concat(array2);
join() : converts array to string.
const elements = ['Fire', 'Air', 'Water']; console.log(elements.join()); // expected output: "Fire,Air,Water" console.log(elements.join('')); // expected output: "FireAirWater" console.log(elements.join('-')); // expected output: "Fire-Air-Water"
sort() : used to sort array
const months = ['March', 'Jan', 'Feb', 'Dec']; months.sort(); console.log(months); // expected output: Array ["Dec", "Feb", "Jan", "March"]
reverse() : used to reverse the array
const array1 = ['one', 'two', 'three']; console.log('array1:', array1);
every() : it testes for each and every element of array whether or not it passed the test.
const isBelowThreshold = (currentValue) => currentValue < 40; const array1 = [1, 30, 39, 29, 10, 13]; console.log(array1.every(isBelowThreshold));
some() : It is used to tests whether at least one element in the array passes the test.
const array = [1, 2, 3, 4, 5]; // checks whether an element is even const even = (element) => element % 2 === 0; console.log(array.some(even));
filter() : It creates a new array with all elements that pass the test
const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present']; const result = words.filter(word => word.length > 6); console.log(result); // expected output: Array ["exuberant", "destruction", "present"]
map() :
const array1 = [1, 4, 9, 16]; // pass a function to map const map1 = array1.map(x => x * 2); console.log(map1); // expected output: Array [2, 8, 18, 32]
reduce() : this method creates a new array populated with the results of calling a provided function on every element in the calling array.
const array1 = [1, 4, 9, 16]; // pass a function to map const map1 = array1.map(x => x * 2); console.log(map1); // expected output: Array [2, 8, 18, 32]
How to empty an array in JavaScript?
By using pop method
const arr = [1,2,3,4];while(arr.length > 0) {
arr.pop();
}
By using splice method
const arr = [1,2,3,4];
arr.splice(0,arr.length)
;
By setting its length to 0
const arr = [1,2,3,4]; arr.length = 0;
By simple reassigning it to empty array
const arr = [1,2,3,4];
arr.length = 0;
Explain forEach loop in JavaScript?
used to traverse each element of array
const apps = ['WhatsApp', 'Instagram', 'Facebook'];
const playStore = [];
apps.forEach(function(item){
playStore.push(item)
});
console.log(playStore);
How to convert array to string?
By using join method
const arr1 = [1,2,3,4,5] arr1.join('');
Explain hoisting in JavaScript.
It means a variable can be declared after it has been used.
x = 5; var x;
What is rest parameter in JavaScript?
The rest parameter syntax used to represent an indefinite number of arguments as an array.
function sum(…theArgs) { return theArgs.reduce((previous, current) => { return previous + current; }); } console.log(sum(1, 2, 3));
What is the difference between let and var?
When variables are declared using let , their scope is limited to locally to that block.
let x = 1; if (x === 1) { let x = 2; console.log(x); //output: 2 } console.log(x); // output: 1
When variables are declared using var ,their scope is global
var x = 1; if (x === 1) { var x = 2; console.log(x); // expected output: 2 } console.log(x); // expected output: 2
So, var is function scoped and let is block scoped.
How to add two numbers as string in JavaScript?
+ operator is used to concatenate two words in JavaScript. So to concatenate plus operator using + first convert theme to number using unary + operator
var num1 = '10',
varnum2 = '10.5';
sum =+num1 + +num2;
So, these are some of the questions that may ask in an interview. Also you can check ES6 interview questions and JavaScript advanced interview questions. You can share your experience and if you like this article please leave feedback.