Interview FAQ PHP Interview FAQ PHP
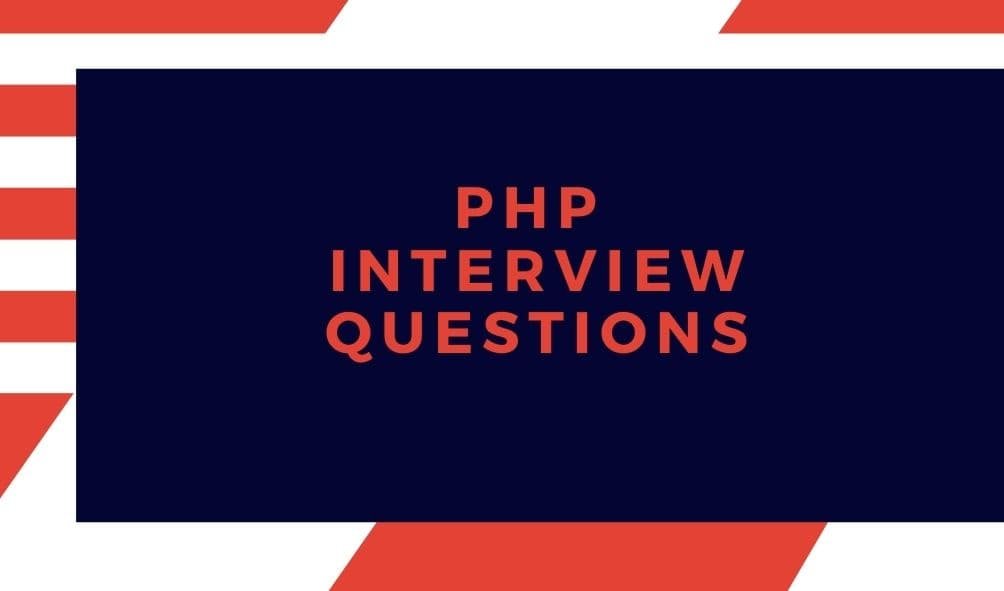
What is PHP?
Hypertext Preprocessor (PHP) is server side scripting language for web development. It was created by Rasmus Lerdorf in 1994. the PHP reference implementation is now produced by The PHP Group
What is the difference between echo & print_r statement?
They both are used to print values. However print_r() is used to print an array,object while echo is used to print only single values like variables, constants etc.
$num_arr = [1,2,3,4]; echo $num_arr; // prints only Array print_r($num_arr); // prints Array(1,2,3,4)
What is the difference between require,require_once(),include,include_once()?
They all are used to include files.
Only difference between require and include is about the error they produce. require() gives fatal error if the file is not found and stop the script execution but include() gives a warning but the script continue to execute.
require() and require_once() are identical but require_once is used to check if the file is already included on same page or not. If it is not included, it includes the file otherwise skip to add those file.
How to access data using GET & POST method in PHP?
In GET method , data is sent via url
<form action = "<?php $_PHP_SELF ?>" method = "GET"> <input type = "text" name = "name" placeholder="Enter Your Name"/> <input type = "text" name = "age" placeholder="Enter Your Age"/> <input type = "submit" /> </form>
value is passed using page_url?name=curious&age=27
this value can be accessed using $_GET[”]
<?php if( $_GET["name"] || $_GET["age"] ) { echo "Name ". $_GET['name']; echo "Age ". $_GET['age']; } ?>
GET method is used when data sent is small like for search, filtration etc. Multimedia can not be sent via GET Method.
POST method : Data is sent via HTTP headers. There is no restriction on the size of data.
<form action = "<?php $_PHP_SELF ?>" method = "POST"> <input type = "text" name = "name" placeholder="Enter Your Name"/> <input type = "text" name = "age" placeholder="Enter Your Age"/> <input type = "submit" /> </form>
<?php if( $_GET["name"] || $_POST["age"] ) { echo "Name ". $_POST['name']; echo "Age ". $_POST['age']; } ?>
How to define Constant in PHP?
using define()
Syntax
<?php define(name, value, case-insensitive); ?>
By default case-sensitive value is false
Example
<?php define("sayHello", "Hello!!"); ?> <?php define("sayHello", "Hello!!", true); ?>
What is cookie ? How to create a cookie in PHP?
Cookie is a small file that is stored at client browser.
To create a cookie in PHP
<?php setcookie(name, value, expire); ?>
Example:
<?php setcookie("Name", "John", time()+1*60*60); ?>
Here time is optional
To get a value of cookie
<?php $_COOKIE["Name"]; ?>
What is a session? How to create a session in PHP?
Session used to stores files on server temporarily. The location of the temporary file is determined by a setting in the php.ini file called session.save_path.
To create a session , first start a session by using session_start()
To set a session
<?php $_SESSION['name'] = 'john'; ?>
Get session value
<?php if( isset( $_SESSION['name'] ) ) { echo $_SESSION['name']; } ?>
Unset Session value
<?php unset($_SESSION['name']); ?>
To destroy all session values
<?php session_destroy(); ?>
How to connect to a database using PHP?
using mysqli_connect()
<?php // connection to the database $servername = "localhost"; $username = "username"; $password = "password"; //object oriented way $conn = new mysqli($servername, $username, $password); // procedural way //mysqli_connect(host, username, password, dbname, port, socket) // Check connection if ($conn->connect_error) { die("Connection failed due to : " . $conn->connect_error); } else{ echo "Connecton Successful"; } ?>
How to delete a file using PHP?
using unlink() function
<?php unlink("test.txt"); ?>
Name PHP string function?
Function Name | Description |
---|---|
trim() | removes white space or other characters from both ends of the string |
explode() | convert strings to array |
implode() | convert array to strings |
join() | join array elements with a string |
ltrim() | trim white space or other characters from left side of the string |
rtrim() | trim white space or other characters from right side of the string |
str_getcsv() | parse csv files into array |
str_repeat() | repeats a string specified number of times |
str_word_count() | count number of words in a string |
stripos() | returns the first occurrence of matched string . It is case sensitive |
strrpos() | returns the last occurrence of matched string . It is case sensitive |
strcmp() | compare two strings. It returns 0 if strings matched. and returns -1 if string1 is less than the string 2. |
strtolower() | converts string to lowercase |
strtoupper() | converts string to uppercase |
substr() | returns part of string |
substr_compare() | compare two strings from a specified start position |
substr_count() | count the occurrence of a sub-string in a string |
substr_replace() | replace a part of string in another string |
Name date function
Function Name | Description |
---|---|
date() | returns local date and time |
date_diff() | compare two dates and return the difference |
date_add() | used to add the days in a date |
strtotime() | parse text date to unix format(number) |
time() | returns current time in unix format |
Name PHP array function?
Function Name | Function Description |
array() | to create array |
array_diff() | find difference between arrays and returns the array of different elements |
array_diff_assoc() | compare associative array |
array_diff_key() | compare key values |
array_key_exists() | check if array key exists |
array_keys() | returns all keys of an array |
array_merge() | merge one or more array into another |
array_push() | push array at the end of the array |
array_reverse() | returns array in reverse order |
array_search() | search for element and returns its key |
array_shift() | remove first element and return its valye |
array_slice() | returns selected part of an array |
array_splice() | used to remove and replace value of an array element |
array_sum() | used to returns sum of array element |
array_unique() | remove duplicate elements from array |
array_unshift() | add elements at the beginning of array |
count() | returns the number of elements in array |
in_array() | check if specific element exist in array() |
is_array() | check if variable is array or not |
krsort() | sort associative array in descending array, according to key |
ksort() | sort associative array in ascending array, according to key |
rsort() | sort indexed array in descending array |
sort() | sort indexed array in ascending array |
What is the difference between session & Cookie?
They both are used to store information. But here is the difference :
Cookie | Session |
Store information on browser | store information on server |
expiration time of cookie is set by the user. | session ends when user close browser |
max cookie size is 4kb. | there is no restriction on the size of session. |
File Upload & Download function in PHP?
To upload a file
<?php move_uploaded_file($file, $dest) ?>
File can be download via different methods
- Using file_get_content()
Here file_get_content first download the file and using file_put_content it saves the file.
<?php file_put_contents( $file_name,file_get_contents($url_of_file))
; ?>
2. Using curl
<?php
// Initialize a file URL to the variable
$url
=
'https://curiouswebs.in/wp-content/uploads/2019/12/women.png';
// Initialize the cURL session
$ch
= curl_init($url);
// Inintialize directory name where
// file will be save
$dir
= './';
// Use basename() function to return
// the base name of file
$file_name
= basename($url);
// Save file into file location
$save_file_loc
= $dir
. $file_name;
// Open file
$fp
= fopen($save_file_loc, 'wb');
// It set an option for a cURL transfer
curl_setopt($ch, CURLOPT_FILE, $fp);
curl_setopt($ch, CURLOPT_HEADER, 0);
// Perform a cURL session
curl_exec($ch);
// Closes a cURL session and frees all resources
curl_close($ch);
// Close file
fclose($fp);
?>
What is an array? How to create an array? What is the difference between indexed, multidimensional and associative array?
An array is a collection of similar items stored in a variable. Array index always starts from 0.
Example of simple array in PHP
<?php $fruits = array("Banana", "Apple", "Orange"); echo $fruits[0], $fruits[0], $fruits[0]."are my favorite fruits." ?>
In PHP, there are three types of arrays.
- Indexed arrays – Arrays with a numeric index
- Associative arrays – Arrays with named keys
- Multidimensional arrays – Arrays containing one or more arrays
Indexed Arrays
<?php $fruits = array("Banana", "Apple", "Orange"); for($i = 0; $i < $arrlength; $i++) { echo $fruits[$i]."<br/>"; } ?>
Associative arrays
<?php $person = array("fname" => "John", "lname", "Cena"); $person['age'] = 35; // add values too array // access values foreach( $person as $key => $value) { echo $key." : ". $value."<br/>; } ?>
Multidimensional Array
$posts = array( array("First Post","lorem ipsum dummy content"), array("Second Post","lorem ipsum dummy content"), array("Third Post","lorem ipsum dummy content") );
How to send email using PHP?
Using php mail() function.
<?php $to = "xyz@yopmail.com"; $subject = "Test Email"; $msg = "This is test email. Kindly ignore"; mail($to,$subject,$msg); ?>
Headers can also be modified
<?php $to = "xyz@yopmail.com, abc@yopmail.com"; $subject = "Test Email"; $msg = "This is test email. Kindly ignore"; $header = "From:abc@somedomain.com \r\n"; $header .= "Cc:afgh@somedomain.com \r\n"; $header .= "MIME-Version: 1.0\r\n"; $header .= "Content-type: text/html\r\n"; mail($to,$subject,$msg,$headers); ?>
What is the method to execute a PHP script from the command line?
using php command
C:\xampp\htdocs>php test.php
What is header()?
It is predefined function in php used to set or modify HTTP headers values
For example to redirect user from one page to another.
<?php
header('Location: http://www.example.com/');
?>
set type
<?php header('Content-Type: application/pdf');
?>
set cache directives
<?php
header("Cache-Control: no-cache, must-revalidate"); // HTTP/1.1
header("Expires: Sat, 26 Jul 1997 05:00:00 GMT"); // Date in the past
?>
What are the encryption functions in PHP?
Fucntion | Description |
crypt() | returns the encrypted string by using DES algorithms. |
encrypt() | encrypts a string using a supplied key. |
base64_encode() | encrypts the string based on 64 bit encryption. |
base64_decode() | decrypts the 64 bit encoded string. |
md5() | Calculates the MD5 hash of the string using RSA data security algorithm. |
Mcrypt_cbc() | Encrypts data in Cipher block chain mode |
mcrypt_encrypt | Encrypts plaintext with given parameters |
How to convert string to array into PHP?
using explode function
<?php explode(' ',"this is a string"); ?>
PHP default constants?
Magical Constants | Description |
__LINE__ | The current line number of the file. |
__FILE__ | The full path and filename of the file |
__FUNCTION__ | The function name, or {closure} for anonymous functions. |
What is PDO?
The PHP Data Objects (PDO) defines a lightweight interface for accessing databases in PHP. It provides a data-access abstraction layer for working with databases in PHP. It defines consistent API for working with various database systems.
<?php $servername = "localhost"; $username = "admin"; $password = "admin_123"; try { $conn = new PDO("mysql:host=$servername;dbname=myDB", $username, $password); // set the PDO error mode to exception $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); echo "Connection success"; } catch(PDOException $e) { echo "Connection failed: " . $e->getMessage(); } ?>
What are super global variables ?
These are PHP predefined variables also called superglobals as they are accessible everywhere i.e. in any class , function, file etc.
$_SESSION | Session variables |
$GLOBALS | References all variables available in global scope |
$_COOKIE | HTTP Cookies |
$_ENV | Environment variables |
$_FILES | HTTP File Upload variables |
$_REQUEST | HTTP Request variables |
What does preg_match(), preg_split() do?
preg_match() function searches string for pattern, returning true if pattern exists, and false otherwise.
<?php
preg_match('/foo/', 'foobarbaz', $matches, PREG_OFFSET_CAPTURE);
print_r($matches);
?>
preg_split() function is used to convert a string into an array.
<?php $ip = "123.456.789.000"; $iparr = preg_split ("/\./", $ip); print_r($iparr); ?>
How will you create class & object in PHP? How to access member functions of a class in PHP?
<?php class Person{ public $fname; public $lname; function set_name($fname, $lname){ $fname = $this->fname; $lname = $this->lname ; } function get_name(){ return $this->fname." ".$this->lname ; } } $person = new Person(); //creating object : instance of class $person.set_name("curious","webs"); // accessing member function echo $person.get_name();
How to create constructor & destructor in PHP?
Constructor : It is used to initialize object properties at the time of creation of object.
<?php class Person{ public $fname; public $lname; function __construct($fname, $lname){ $fname = $this->fname; $lname = $this->lname ; } function get_name(){ return $this->fname." ".$this->lname ; } } $person = new Person( "curious","webs"); echo $person.get_name();
Destructor : It is used to destruct the object properties. This function is automatically called at the end of the script.
<?php class Person{ public $fname; public $lname; function __construct($fname, $lname){ $fname = $this->fname; $lname = $this->lname ; } function __destruct(){ echo $this->fname." ".$this->lname ; } } $person = new Person( "curious","webs");
What is meant by public, private, protected, static and final scopes?
public : the property or method can be access from anywhere. By default properties and methods are public.
protected : the property or method can e accessible by class itself or classes inherited from it.
private : only member of the class can access its data.
<?php class Person { public $name; protected $age; private $sex; } $person = new Person(); $person ->name = 'John'; $person ->age = '30'; // fatal error $person ->sex = 'Female'; // fatal error ?>
What is inheritance?
The process of acquring parent class properties into child class is called inheritance.
Using extend keyword
<?php
class Vehicle {
// parent class code
}
class Car extends Vehicle {
// child class code
}
class Ford extends Vehicle {
// child class code
}
?>
Click here more info on PHP classes and inheritance.
Does PHP supports multiple inheritance? What about multilevel inheritance?
PHP does not support multiple inheritance. PHP supports multileve inheritance. Child class class can’t inherit by more than one parent class.
class A { // body } class B { // body } class C extends B { // body } // will not work
//Multilevel Inheritance class A { // body } class B extends A { // body } class C extends B { // body }
What is the use of final keyword?
If a function is defined with final keyword it can not be override. Same if class is declared final itself , it cannot be inherited to some another class.
<?php
class BaseClass {
public function test() {
echo "BaseClass::test() called\n";
}
final public function moreTesting() {
echo "BaseClass::moreTesting() called\n";
}
}
class ChildClass extends BaseClass {
public function moreTesting() {
echo "ChildClass::moreTesting() called\n";
}
}
// Results in Fatal error: Cannot override final method BaseClass::moreTesting()
?>
What is function overloading and overriding?
Function Overloading : When two or more function have same name but different number of arguments is called function overloading.
This can be achieved in PHP via _call() magic method that takes function name and arguments
<?php class Person{ function _call($func_name,$arguments){ if($func_name == "name"){ switch(count($arguments)){ case 0 : return; case 1 : return $arguments[0]; case 2 : return $arguments[0] . " " . $arguments[1]; } } } } $person = new Person(); echo $person->name("curious"); echo $person->name("curious","webs");
Function Overriding : It is used to override parent class function. In this both parent and child class have same function name and same number of arguments.
class Person{ public function name(){ echo "John"; } } class Male extends Person(){ public function name(){ echo "John"." Cena"; } $person = new Person(); $person->name(); // John $male = new Male(); $male->name(); // John Cena }
How can a cross-site scripting attack be prevented by PHP?
XSS attacks can be prevented by
1) Data Validations : By proper validating the data. For example password must be at least 8 character long, having one special character, capital letter.
2) Data Sanitization : Data can be sanitized before storing into the database. For example for name value make sure there is no html tag associated with it. Using strip_tags
remove all html tags from it.
For more information about xss attacks check this article here.
What is the use of imagetypes() method?
returns the type of image.
<?php
if (imagetypes() & IMG_PNG) {
echo "PNG Support is enabled";
}
?>
Explain type casting and type juggling.
The way by which PHP assign type to its variable is called type casting.
$str = "10"; //type is string $str = (boolean) $str; // type is now boolean
Now, a variable’s type is determined by the context in which the variable is used. So, the type of the variable is changed automatically based on the value assigned to it. This is called Type juggling.
What is meant by ‘passing the variable by value and reference’ in PHP?
By default variables are passed by value in PHP. However to pass by reference i.e. to make changes in argument use & sign before the variable.
<?php function add_some_extra(&$string) {
$string .= ' adding extra string';
}
$str = 'Hello';
add_some_extra($str);
echo $str;
?>
What is php.ini file ?
php.ini file contains php configuration settings. This file is located at the root of site. Each time PHP is initialized, the php.ini file is read by the system.
Some Basic Settings in php.ini file
- memory_limit
- upload_max_filesize
- post_max_size
- display_errors
- error_reporting
- max_execution_time
- file_uploads
- mysql.default_host
- mysql.default_user
- mysql.default_password
- doc_root
- include_path
Is PHP a strongly typed language?
No, PHP is not strongly typed language. It is loosely type language i.e variable declaration does not required before using it. On the other hand like C# are strongly typed language, a variable must be declared before using.
What is the difference between isset() and empty()?
empty returns true if the variable is empty i.e. ” “(empty string). But isset() checks whether the value is set ot not. It returns true if value is set other than NULL.
What does var_dump() do?
It is used to dump information about the variables. It display variable’s type and value.
How to add comments in PHP?
For single line
/** *** Multi line comment **/ $a = 10; // single line comments
Why enctype=”multipart/form-data” is used?
HTML5 provides three methods of encoding
- application/x-www-form-urlencoded(default)
- multipart/form-data : this is used to upload files to the server. It means no characters will be encoded. So It is used when a form requires binary data, like the contents of a file, to be uploaded
- text/plain
How many types of errors are there in PHP?
- Fatal Error : Fatal error stops the execution of the php script.
- Syntax Error : If there is mistake in php syntax.
- Warning Errors : These are just warnings and usually occur for undefined constants, incorrect number of parameters.
- Notice Errors : They generally does not stop the execution of the script.
When is the difference between break and continue statement?
break statement is used to terminate a block and gain control out of the loop or switch, whereas continue statement will not terminate the loop but promotes the loop to go to the next iteration.
continue statement can not be implemented in switch. break statement can be used in any for, while, do-while, switch statement
What is the difference between abstract class and interface in php?
Abstract | Interface |
Abstract methods can declare with protected, public, private | Interface methods stated with the public. |
can have method stubs, constants, members, and defined processes | interfaces can only have constants and methods stubs. |
do not support multiple inheritance | interface support multiple inheritance |
can contain constructors | does not support constructors. |
What is namespace in PHP?
Namespace are used to define same class name or function name in one program.
<?php
namespace
MyNamespaceName;
function
hello()
{
echo
'Hello I am Running from a namespace!';
}
// Resolves to MyNamespaceName\hello
hello();
// Explicitly resolves to MyNamespaceName\hello
namespace\hello();
?>
How to get current page or filename in PHP?
By using $SERVER[“PHP_SELF]
What is php curl used for?
It is php library used to download data over FTP or HTTP.
$ch = curl_init("
https://curiouswebs.in"); $fp = fopen("index.php", "w"); curl_setopt($ch, CURLOPT_FILE, $fp); curl_setopt($ch, CURLOPT_HEADER, 0); curl_exec($ch); curl_close($ch); fclose($fp);
What is the difference between $$var and $var.
$$var is a normal variable. $$var is a reference variable