SQL Interview FAQ Interview FAQ
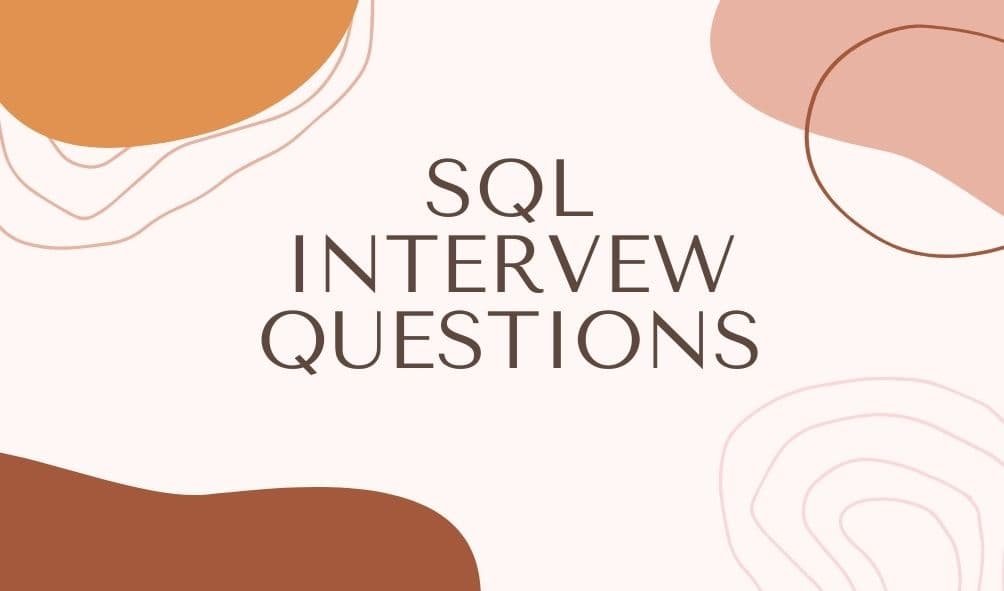
What is database?
Database is an organized collection of data stored electronically.
What is database management system?
A database management system(DBMS) is a software which is used to manage database. It allows to perform a number of operation(CURD) on database while considering appropriate security measures.
What is relational database?
A relational database is a collection of data items with pre-defined relationships between them. A relational database is based on relational model.
Relational Modal organizes data into two or more tables. Each table has set of columns and rows with a unique key identifying each row.
Rows are called records or tuples. Columns are called attributes.
Example: MySQL, PostgresSQL, MariaDB, Microsoft SQL Server.
What is SQL?
Structured Query Language is used to write queries for manipulation to the database. It is used to manage RDBMS and used to perform various operations(CRUD) etc. on it.
What is NoSQL database?
NoSQL systems use JSON format to store data.
Example : MongoDB, CoucheDB etc
NoSQL databases are a great fit for many modern applications such as mobile, web, and gaming that require flexible, scalable, high-performance, and highly functional databases.
Is SQL case sensitive language?
No, SQL is not case sensitive language. But often SQL syntax are written in uppercase.
Syntax of SELECT Query?
Select everything from a table name books
SELECT * FROM `books
`;
What is clause in SQL query?
clause are used to filter result from the database
Clause | Description |
FROM Clause | Used to list out tables and join information |
WHERE Clause | Used to filter results |
DISTINCT Clause | Used to get unique results |
GROUP BY Clause | Used to group by one or more columns |
HAVING Clause | Used to restrict the groups of returned rows |
ORDER BY Clause | Used to sort the query results |
What is alias in SELECT query?
SQL SELECT AS alias is used to assign temporary names to a table or column.
- Advantages of using SQL alias
- increase the speed of query as it replace complex and long name with shorter and simpler one..
- protect the column names of the databases by not showing the real column names.
SELECT column_name1 AS alias_name1, column_name2 AS alias_name2, ... FROM table_name;
How to remove duplicate record from select query?
Using DISTINCT keyword.
For example to select unique name from student table
SELECT DISTINCT student_name FROM student;
Give an example of IN operator
IN operator is a logical operator in SQL used to test whether specific value matches in a given list. It is an alternative of OR operator.
SELECT * FROM Products WHERE price IN ('200', '300', '400');
BETWEEN operator in SQL?
The BETWEEN operator is logical operator that is used to select records from a given range of values.
SELECT * FROM Products WHERE price BETWEEN 100 AND 200;
LIKE operator in SQL?
LIKE operator is used to find records that matches with the specified pattern
SELECT * FROM Students WHERE student_name LIKE 'm%';
What kind of REGEXP used in SQL query?
% | zero or more number of characters |
? | only single character |
[] | any single character withing the bracket |
! | not matched with the specified pattern |
# | any single numeric character |
– | range of characters |
^ | any character not in the brackets |
What does IS NULL operator do?
It is used to check whether the value is null or not.
SELECT address FROM Student WHERE address IS NULL;
Similarly IS NOT NULL operator can be used.
SELECT address FROM Student WHERE address IS NOT NULL;
What is primary key?
Primary key has two properties.
- It must be unique
- It can not be null
A tale can have only one primary key.
LIMIT and OFFSET in Query?
The LIMIT keyword is used to limit the number of rows returned by SQL query.
SELECT * FROM Products LIMIT 20;
This will return 20 records from the table. Now to select records from 21-40, OFFSET can be used in SQL query.
SELECT * FROM Products LIMIT 20 OFFSET 21;
This can also be shortened like
SELECT * FROM Products LIMIT 20, 21
How to get second highest salary from employee table?
Using Limit to find second highest salary from employees table
SELECT * FROM employees ORDER BY salary DESC LIMIT 1 OFFSET 1;
Without using limit clause
SELECT MAX(salary) FROM employees WHERE salary NOT IN(SELECT MAX(salary) FROM employees);
using < operator
SELECT MAX(salary) FROM employees WHERE salary < (SELECT MAX(salary) FROM employees);
What is Inner join?
SQL Inner join returns the results that are common in both the tables.
SELECT * FROM table1 INNER JOIN table2 ON table1.column_name = table2.column_name;
SELECT * FROM table1 JOIN table2 ON table1.column_name = table2.column_name;
What is foreign key in SQL?
A foreign key is a column or set of column in a table whose value depends upon primary key of another table.
Example to create foreign key for Customer_orders tables that depends on Id (primary key) of customer table
CREATE TABLE Customer_orders( ID INT NOT NULL, DATE DATETIME, CUSTOMER_ID INT references customers(ID), AMOUNT double, PRIMARY KEY (ID) );
How to join multiple table from different databases?
just add database name before the column or table name.
SELECT db1.customer.name, db2.product.name FROM db1.customer INNER JOIN db2.product ON db1.customer.id = db2.product.id;
What is self join?
When a table join with itself is called self join.
SELECT * FROM table1 a, table1 b WHERE condition;
What is composite primary key?
Composite key is set of more than one column that makes primary key. It can have at most one primary key.
Explain outer joins?
OUTER JOIN : It returns the unmatched rows from both the table.
LEFT OUTER JOIN : It returns all the records from first table and matched records in table two.
SELECT columns FROM table1 LEFT JOIN table2 ON table1.column_name = table2.column_name
SELECT * from employees LEFT JOIN offices ON employees.office_id = offices.office_id
RIGHT OUTER JOIN : It returns all the records from second table and matched record in the first table
SELECT columns FROM table1 RIGHT JOIN table2 ON table1.column_name = table2.column_name
SELECT * from employees LEFT JOIN offices ON employees.office_id = offices.office_id
What is EQUI JOIN?
An EQUI JOIN is a join with a join condition containing an equality operator. It returns only the rows that have equivalent values for the specified columns
What is USING clause?
The USING clause is used to match only one column when more than one column matches. It is used to specify to specify the columns for the EQUI JOIN where several columns have the same names but not same data types. The NATURAL JOIN and USING clauses are mutually exclusive .
SELECT first_name, address FROM employees JOIN offices USING (office_id)
What is NATURAL JOIN?
It is type of EQUI JOIN and is structured in such a way that, columns with the same name of associated tables will appear once only . Here the matched column data type must be same. Do not use ON clause in Natural join.
SELECT * FROM employees NATURAL JOIN offices
What is cross join?
The cross join returns result set in which the number of rows in the first table multiplied by the number of rows in second table. It is more like Cartesian product if no where clause is used. With where clause it is same like INNER JOIN
SELECT * FROM employees CROSS JOIN offices
How to Insert values into a table?
INSERT INTO table_name (column1, column2, column3, ...) VALUES (value1, value2, value3, ...);
What does LAST_INSERT_ID() function do?
This function returns the AUTO_INCREMENT id of the last row that has been inserted or updated in a table.
SELECT LAST_INSERT_ID();
Write the syntax and example of create table command?
CREATE TABLE your_table_name ( column_name1 datatype, column_name2 datatype, .... );
CREATE TABLE employee( id int, employee_name varchar(25), address varchar(255) );
How to copy a table into another table?
Using SELECT INTO statement
SELECT * INTO table1 FROM table2
How to update data in SQL?
UPDATE table_name SET column1 = value1, column2 = value2, ... WHERE condition;
UPDATE employee SET employee_name = "Jass" WHERE id = 1;
What is subquery in SQL?
A subquery is a query within the query. A subquery must be enclosed within parenthesis.
subquery in SELECT Statement
SELECT * FROM sql_hr.employees WHERE employee_id IN (SELECT employee_id FROM sql_hr.employees WHERE SALARY > 4500) ;
How to delete data in SQL?
DELETE FROM table_name WHERE column1 = value;
DELETE FROM employee WHERE id = 1;
What are aggregate functions in SQL?
Function | Description | Example |
MAX() | Used to find max value from the column | SELECT MAX(salary) FROM employees; |
MIN() | Used to find minimum value from the column | SELECT MIN(salary) FROM employees; |
AVG() | returns the average value of numeric column | SELECT AVG(salary) FROM employees; |
COUNT() | returns the number of rows that matched to the specified criteria | SELECT COUNT(employee_id) FROM employees; |
SUM() | returns the sum of a numeric column | SELECT SUM(salary) FROM employees; |
MOD() | returns the remainder | SELECT MOD(25, 3); |
What is GROUP BY clause?
GROUP BY clause is used to group results on the basis of one or more columns.
SELECT * FROM sql_hr.employees WHERE office_id < 4 GROUP BY salary
What is HAVING clause?
HAVING clause is used to find results that matches with specific value.
SELECT * FROM sql_hr.employees WHERE office_id < 4 GROUP BY salary HAVING employee_id < 37851
What is ROLLUP command?
The ROLLUP command is used to include extra rows along with the total of rows.
SELECT * FROM sql_hr.employees GROUP BY salary, office_id WITH ROLLUP
What is the difference between subquery and JOIN in SQL?
They both are used to combine data from different tables into single result. JOINS are usually faster than subquery. Subquery have more readabilityas compared to joins.
What is ALL keyword?
ALL keyword is used with WHERE and HAVING clause. IT returns true if all the sub query value matches the condition.
SELECT * FROM employees WHERE office_id = ALL (SELECT office_id FROM offices WHERE state = "VA");
What is any/some keyword?
They both are used with WHERE and HAVING clause.
ANY : returns true if any of sub query matches the value.
SOME : returns true if some of sub query matches the value.
SELECT * FROM employees WHERE office_id = ANY (SELECT office_id FROM offices WHERE state = "OH");
SELECT * FROM employees WHERE office_id = SOME (SELECT office_id FROM offices WHERE state = "OH");
What does Exist Operator do?
It returns true if the sub query returns one or more results.
SELECT * FROM employees WHERE EXISTS (SELECT office_id FROM offices WHERE state = "OH");
How to write subquery in select clause?
SELECT first_name FROM sql_hr.employees WHERE office_id IN (SELECT office_id FROM sql_hr.offices WHERE state = "NY")
How to use subquery in from clause?
SELECT i.first_name,i.last_name FROM ( SELECT office_id FROM sql_hr.offices WHERE state = "OH") AS STATE , sql_hr.employees AS i WHERE i.office_id = STATE.office_id;
ROUND() function in SQL?
It is used to round values up-to a digit.
SELECT ROUND(160.896, 3);
Name some numeric function in SQL?
Name | Description | Example |
TRUNCATE() | used to truncate value | SELECT TRUNCATE(180.0987, 2); |
CIELING() | round off to largest nearest integer value | SELECT CEILING(25.75); |
FLOOR() | round off to smallest integer value | SELECT FLOOR(25.75); |
ABS() | returns positive value | SELECT ABS(-10); |
Name SQL string functions.
Function | Description | Example |
LENGTH() | used to find length of string | SELECT LENGTH(“Curious Webs”) AS StringLength; |
UPPER() | used to convert into uppercase letters | SELECT UPPER(“Curious webs”); |
LOWER() | used to convert into uppercase letters | SELECT LOWER(“Curious webs”); |
TRIM() | used to trim spaces from both ends of the string | SELECT TRIM(” Curious webs “); |
RTRIM() | used to trim space at the end of string | SELECT RTRIM(” Curious webs “); |
LTRIM() | used to trim space at the beginning of string | SELECT LTRIM(” Curious webs “); |
LEFT() | used to extract letters from the left side of string | SELECT LEFT(“Curious Webs”, 5); |
RIGHT() | used to extract letters from the right side of string | SELECT RIGHT(“Curious Webs”, 3); |
SUBSTRING() | used to extract substring | SELECT SUBSTRING(“Curious Webs”, 9, 3); |
LOCATE() | returns the position of string | SELECT LOCATE(“ou”, “Curious Webs”); |
REPLACE() | used to replace string | SELECT REPLACE(“Curious Webs”, “Webs”, “Panda”); |
CONCAT() | used to comine more than one string | SELECT CONCAT(“curious “, “webs”); |
MID() | used to extract sub string from a given position | SELECT MID(“Curious”, 2, 2) ; |
REPEAT() | repeat a string for given number of times | SELECT REPEAT(“curious”, 2); |
SPACE() | returns string with space character | SELECT SPACE(10); |
POSITION() | returns the position of string | SELECT POSITION(“ou”, “Curious Webs”); |
How to get current date & current time in SQL?
Using GETDATE() function.
SELECT GETDATE();
Name some SQL DATE function.
Function | Description | Example |
YEAR() | return year from the date | SELECT YEAR(‘2020/05/25’); |
DAY() | return the day of the month from a date | SELECT YEAR(‘2020/05/25’); |
HOUR() | return hour from time | SELECT HOUR(“04:35”) |
MINUTE() | return minute | SELECT MINUTE(“04:35”) |
SECOND() | return second | SELECT SECOND(“04:35:2”) |
DAYNAME() | return dayname from date | SELECT DAYNAME(“2020-05-21”) |
MONTHNAME() | return month name from string | SELECT MONTHNAME(“2020-05-21”) |
EXTRACT() | extract month from a date | SELECT EXTRACT(MONTH FROM “2021-05-21”); |
DATE_FORMAT() | used to format a date | SELECT DATE_FORMAT(“2021-05-21”, “Year : %Y”); |
DATE_ADD() | used to add date in a given date | SELECT DATE_ADD(“2021-05-21”, INTERVAL 5 DAY); |
DATE_SUB() | used to subtract days from a given date | SELECT DATE_SUB(“2021-05-21”, INTERVAL 5 DAY); |
DATEDIFF() | used to find difference between two days | SELECT DATEDIFF(“2021-08-15”, “2021-05-15”); |
What is the difference between ISNULL() and COALESC() function?
ISNULL() function take two arguments whereas there is no limit on number of arguments inn COALESC() function. In COALESC() function arguments must be of same data type.
SELECT IFNULL(NULL, "W3Schools.com");
IF function in SQL?
this function checks the condition if it is true returns true otherwise false.
SELECT IF(1200<10, "TRUE", "FALSE");
CASE operator in SQL?
This is used to to check for multiple cases
SELECT first_name, salary, CASE WHEN salary > 40000 THEN 'Salary Greater than 10000' WHEN salary < 40000 THEN 'Salary less than 10000' ELSE 'The quantity is under 30' END AS Salary FROM sql_hr.employees;
What is views? advantages of views?
Views are virtual tables based on the SQL select statement.
Advantages of Views : They always shows the updated data. Views hide the complexity of the data. They are used for security purpose as to the database users views are just real tables
How to create,drop view?
To create a view
CREATE VIEW MYVIEW AS SELECT first_name, last_name FROM employees WHERE office_id = 1;
To drop View
DROP VIEW MYVIEW;
What is CHECK Clause in SQL?
CHECK Clause is used to check condition when the value is being entered into table.
CREATE TABLE employee_info( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, AGE INT NOT NULL ADD CONSTRAINT check_age CHECK (AGE >= 18), ADDRESS CHAR (25) , SALARY DECIMAL (18, 2), PRIMARY KEY (ID) );
Add CHECK constraint after table being created
ALTER TABLE employee_info MODIFY AGE INT NOT NULL CHECK (AGE >= 18 );
To remove check constraint
ALTER TABLE employee_info DROP CONSTRAINT check_age;
What are stored procedures?
Stored procedures are set of SQL statements that can be stored, reused and shared by multiple programs.
How to create stored procedure ? Structure/body of stored procedure?
To create a stored procedure
DELIMITER $$ CREATE PROCEDURE emp_detail() BEGIN SELECT * FROM employees; SELECT * FROM offices; END $$ DELIMITER ;
$$ or // can be used
How to execute store procedure in SQL?
call sql_hr.emp_detail();
EXEC procedure_name;
EXECUTE procedure_name;
Drop a store procedure
DROP PROCEDURE sql_hr.emp_detail;
What is the difference between truncate and drop command in SQL?
Both commands are used for deletion operation.
DROP command is used to drop a table or database. If table is droped, this will no longer be available in database.
DROP TABLE tablename; DROP DATABASE databasename;
TRUNCATE command is used to delete a table but it keeps the table structure. It just empty the table means delete all its data.
TRUNCATE TABLE tablename;
TRUNCATE command is usually faster.
How to create Functions?
DELIMITER $$ CREATE FUNCTION emp_grade( salary DECIMAL(10,2) ) RETURNS VARCHAR(20) DETERMINISTIC BEGIN DECLARE empGrade VARCHAR(20);IF salary > 50000 THEN
SET empGrade = 'GROUP 1';
ELSEIF (salary >= 50000 AND salary <= 100000) THEN
SET empGrade = 'GROUP 2';
ELSEIF salary < 10000 THEN
SET empGrade = 'GROUP 3';
END IF;
RETURN (empGrade);
END$$ DELIMITER ;
calling a function
SELECT first_name, emp_grade(salary) FROM sql_hr.employees ORDER BY first_name
drop function
DROP FUNCTION CustomerLevel;
What is trigger?
Trigger is a stored procedure that runs automatically when specific event is fired. For example when student marks is entered into the database their marks total is automatically calculated.
How to create and drop trigger?
create trigger [trigger_name] [before | after] { insert | update | delete } on [table_name] [for each row] [trigger_body]
DROP TRIGGER trigger_name;
Name some JSON function in SQL.
JSON_ARRAY() | used to convert list values into json array | SELECT JSON_ARRAY(1,””,NULL,”house”) AS RESULT; |
What is Candidate key in SQL?
Candidate key is set of one or more than one column having unique values. Every table have at least one candidate key. Candidate key is eligible for primary key. A table has more than one candidate key but primary key will be only one.
What are indexes? How to create and view indexes?
indexes are used to retrieve data from SQL in a speedy way.
CREATE UNIQUE INDEX index_name on table_name (column_name);
CREATE INDEX index_name on table_name (column1, column2);
DROP INDEX index_name;
How to grant or revoke privileges from user?
REVOKE is used to revoke permission from user on tables
REVOKE insert, update ON customer FROM abc@localhost;
GRANT is used to give permission to user on tables
GRANT insert, update ON customer TO abc@localhost;