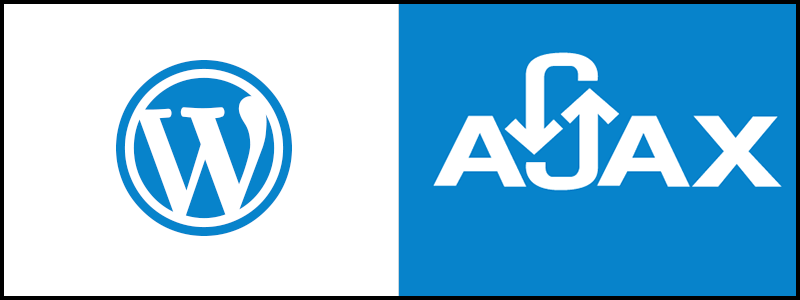
Ajax is “Asynchronous JavaScript and XML“. Basically AJAX helps to update page data without reloading it.
So, this article explain how to use ajax in custom theme with minimal code.
How to use AJAX in custom theme
We have a button. On click of this button we will call data via ajax in this div.
Step 1 : First of all include ajax file in theme. In header.php use
<script type="text/javascript"> var ajaxurl = "<?php echo admin_url('admin-ajax.php'); ?>"; </script>
Explanation : Why we use admin-ajax.php ? and How exactly this admin-ajax.php works?
It is because wordpress default AJAX file stored in wp-admin area. It means WordPress Core is loaded and available to use. So now gloal variables example$wpdb
and $WP_Query
are available to use. It also fires the hooks that user is not logged in.
Find here more details about admin-ajax.php
Step 2 : In template or post page
<div class="load-content-via-ajax" id="load-content-via-ajax"> <button id="button" class="button"></button> </div>
Exaplanation: so data will be loaded into that div having id load-content-via-ajax.
Step 3 : write script for ajax so in footer.php
<script> jQuery(document).ready(function ($) { var $ = jQuery; $("#button").on("click", function () { $this = $(this); // mention wordpress action name here var str = '&action=loadcontent'; $.ajax({ data: str, dataType: "html", type: 'post', //method of action post or get url: ajaxurl, beforeSend: function () { //code to do before ajax is called. }, success: function (data) { //div to load ajax data $("#load-content-via-ajax").html(data); } }); }); // end button click function }); // end jQuery ready function </script>
Exaplanation : on click of the button loadcontent action will be called.
Step 4 : in functions.php
<?php // name must be same as passed in action function loadcontent() { echo "<h2>Hello World!!</h2>"; die(); //otherwise it returns 0 also } add_action('wp_ajax_nopriv_loadcontent', 'loadcontent'); add_action('wp_ajax_loadcontent', 'loadcontent');
Explanation :
wp_ajax_nopriv_actionName and wp_ajax_actionName both are wordpress action hooks.wp_ajax_nopriv_actionName fires non-authenticated Ajax actions for logged-out users .
For reference click here
wp_ajax_actionName : It handles the custom ajax for frontend.It fires only for logged in users.
Learn More about wp_ajax here
To allow both need to define both the hooks.
so this is how AJAX can be used in wordpress custom theme. Well this is simple example. AJAX can be used in wordpress for number of reasons like loading number of posts